Exploring Behavioral Design Patterns in Golang
Written on
Chapter 1: Introduction to Behavioral Design Patterns
This article examines some of the most frequently utilized behavioral design patterns, such as Command, Chain of Responsibility, State, Observer, Memento, Iterator, Interpreter, Strategy, Visitor, Template, and Mediator.
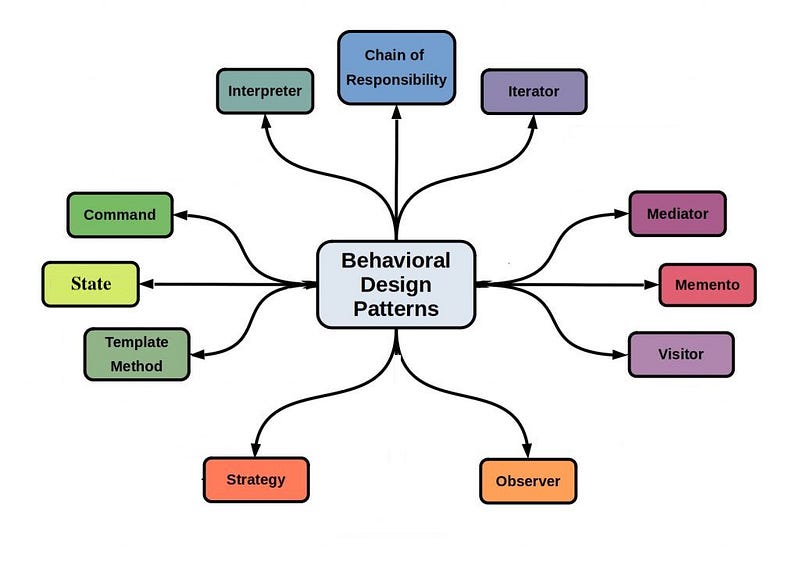
Behavioral design patterns focus on the algorithms and how responsibilities are assigned among objects. These patterns deal with the interactions between objects and their behavior, outlining how they communicate and operate. Key behavioral design patterns include Chain of Responsibility, Command, Interpreter, Iterator, Mediator, Visitor, Memento, Strategy, and others.
Section 1.1: Strategy Pattern
The Strategy pattern is a behavioral design pattern that enables the definition of a family of algorithms, allowing for dynamic switching between them during runtime. This pattern promotes algorithm independence from the clients that utilize them, making it one of the most comprehensible behavioral patterns.
For more insights, please refer to the following video:
Section 1.2: Chain of Responsibility Pattern
The Chain of Responsibility pattern allows various objects to handle a request, giving each object the option to either process the request or pass it to the next object in the chain. This pattern facilitates easy addition or removal of objects in the chain, as the objects interact through a shared interface.
Section 1.3: Command Pattern
The Command pattern encapsulates a request as an object, transforming requests or operations into stand-alone objects that can be stored, passed, and executed independently of the original requester. This separation between the client and the receiver allows for functionalities like passing requests as method arguments and supporting undoable operations.
Section 1.4: Template Pattern
The Template pattern outlines the framework of an algorithm in a base class, permitting subclasses to customize specific steps without altering the overall structure. This design encourages a clear definition of algorithm steps while allowing flexibility in subclass behavior.
Section 1.5: Memento Pattern
The Memento pattern enables an object to save its internal state for future restoration while upholding encapsulation. This method of capturing an object's current state allows for easy restoration without compromising the object's integrity.
Chapter 2: Additional Patterns
The second video provides a concise overview of the top three behavioral design patterns you should be familiar with, offering practical insights into their applications.
Section 2.1: Iterator Pattern
The Iterator pattern provides a means to traverse a collection of objects without exposing the underlying implementation. This enables sequential access to collection elements, allowing users to navigate through them while remaining unaware of the internal structure.
Section 2.2: Interpreter Pattern
The Interpreter pattern is often employed in scenarios requiring a specialized language for executing common operations. It involves creating a set of algorithm classes to address logical challenges.
Section 2.3: Visitor Pattern
The Visitor pattern facilitates the addition of new algorithms to a structure of objects without modifying their classes. By defining a separate visitor object, this pattern allows operations to be performed on various objects within a complex structure.
Section 2.4: State Pattern
The State pattern permits an object to modify its behavior based on its internal state. This design allows behavior changes without altering the object's class, fostering loose coupling and enhancing code maintainability.
Section 2.5: Mediator Pattern
The Mediator pattern streamlines communication among different objects through a central mediator, reducing direct coupling between them. This approach encapsulates the communication logic, allowing for more manageable interactions.
Section 2.6: Observer Pattern
The Observer pattern establishes a subscription mechanism that notifies multiple objects about events affecting the observed object. Also known as the publisher/subscriber pattern, it facilitates state change notifications among objects.
Conclusion
Behavioral patterns emphasize the interactions between objects, enhancing communication and collaboration. They empower developers to craft effective, adaptable, and maintainable code that responds to evolving business needs. Examples of these patterns include Chain of Responsibility, Command, Interpreter, Iterator, Mediator, Memento, Observer, State, Strategy, Template, and Visitor.
These pattern categories provide developers with established solutions to common design challenges, enabling the creation of high-quality, maintainable, and scalable code that can adjust to changing requirements over time.
For further exploration of design patterns in Golang, refer to the links below:
- Creational Design Patterns: [Link]
- Structural Design Patterns: [Link]
- Behavioral Design Patterns: [Link]
- Concurrency Design Patterns: [Link]
- Microservices Design Patterns: [Link]