Unlocking the Power of JavaScript Arrays: A Comprehensive Guide
Written on
Chapter 1: Introduction to JavaScript Arrays
Arrays serve as one of the core data structures in JavaScript, enabling the storage of multiple values within a single variable. Whether you’re just starting out or seeking to strengthen your existing knowledge, mastering arrays is a vital skill for every JavaScript programmer. In this guide, we will delve into the fundamental concepts of arrays in JavaScript, accompanied by practical examples to facilitate your learning.
What Exactly are Arrays?
Arrays are essentially collections of items, each accessible via an index. In JavaScript, these arrays can accommodate various data types, including numbers, strings, objects, and even other arrays. They offer an efficient means to organize and manipulate data.
Creating Your First Arrays
In JavaScript, arrays can be instantiated using the array literal syntax, which involves square brackets [ ]. Here’s a simple way to create an array:
// Creating an array of numbers
let numbers = [1, 2, 3, 4, 5];
// Creating an array of fruits
let fruits = ["apple", "banana", "orange"];
// Creating an array with mixed data types
let mixedArray = [1, "hello", true];
You can also start with an empty array and add elements later:
let emptyArray = [];
emptyArray.push(10); // Appends 10 to the end
emptyArray.push(20);
Accessing Elements in Arrays
You can retrieve elements from an array using their index, keeping in mind that JavaScript array indices begin at 0. Here’s how to access elements:
let numbers = [10, 20, 30, 40, 50];
console.log(numbers[0]); // Output: 10
console.log(numbers[2]); // Output: 30
You can also update elements by their index:
let numbers = [10, 20, 30, 40, 50];
numbers[1] = 25;
console.log(numbers); // Output: [10, 25, 30, 40, 50]
Utilizing Array Methods
JavaScript offers a variety of built-in methods for effective array manipulation. Here are some frequently used methods:
- push(): Appends one or more elements to the end of an array.
- pop(): Removes the last element from an array.
- shift(): Eliminates the first element from an array.
- unshift(): Adds one or more elements to the start of an array.
- slice(): Produces a shallow copy of a segment of an array into a new array.
- splice(): Alters the contents of an array by removing or replacing existing elements.
Let’s look at a few examples:
let numbers = [1, 2, 3];
numbers.push(4); // Adds 4 to the end
console.log(numbers); // Output: [1, 2, 3, 4]
numbers.pop(); // Removes the last element (4)
console.log(numbers); // Output: [1, 2, 3]
numbers.unshift(0); // Adds 0 to the beginning
console.log(numbers); // Output: [0, 1, 2, 3]
let slicedArray = numbers.slice(1, 3); // Retrieves a portion of the array
console.log(slicedArray); // Output: [1, 2]
numbers.splice(1, 2); // Removes elements starting from index 1
console.log(numbers); // Output: [0, 3]
Conclusion: The Importance of Arrays
Grasping the concept of arrays is essential for JavaScript developers. They provide a flexible way to handle collections of data efficiently. By mastering arrays and their associated methods, you can write cleaner and more organized code. Experiment with the examples given and continue to explore further to enhance your understanding of JavaScript arrays.
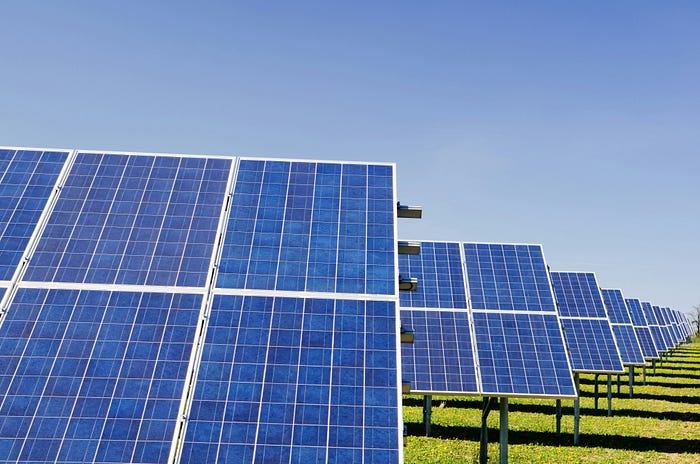
Chapter 2: Practical Insights and Resources
To deepen your understanding of JavaScript arrays, consider the following resources:
Discover advanced techniques and best practices in array manipulation with the following video:
This video titled "JavaScript Array Mastery: Tips, Tricks & Best Practices" provides valuable insights for developers looking to enhance their skills.
For beginners, the following tutorial offers a solid foundation in working with arrays:
The video "Javascript Arrays | Javascript Tutorial For Beginners" serves as an excellent starting point for those new to the language.