Mastering IndexedDB with Dexie: A Guide to Data Manipulation
Written on
Chapter 1: Understanding IndexedDB and Dexie
IndexedDB serves as a method for storing data directly within the browser, allowing for significantly larger storage capacities compared to local storage, all handled asynchronously. Dexie simplifies the process of interacting with IndexedDB.
Section 1.1: Iterating Through Unique Keys
To iterate through unique or primary keys, we can utilize the eachUniqueKey function. Here’s a practical example:
const db = new Dexie("friends");
db.version(1).stores({
friends: "id, name, age"
});
(async () => {
await db.friends.put({ id: 1, name: "jane", age: 78 });
await db.friends.put({ id: 2, name: "mary", age: 76 });
await db.friends
.orderBy('name')
.eachUniqueKey(name => console.log(name));
})();
In this snippet, we call eachUniqueKey following an orderBy on the 'name' field to retrieve the names from each entry.
Section 1.2: Filtering Data
Objects within a collection can be filtered using the filter method. For instance:
const db = new Dexie("friends");
db.version(1).stores({
friends: "id, name, age"
});
(async () => {
await db.friends.put({ id: 1, name: "jane", age: 78 });
await db.friends.put({ id: 2, name: "mary", age: 76 });
const someFriends = await db.friends
.orderBy('name')
.filter(({ age }) => age > 76)
.toArray();
console.log(someFriends);
})();
Here, we add two entries and then apply a filter to return friends who meet the specified age condition.
Section 1.3: Retrieving the First Item
To fetch the first item from a collection, the first method is employed:
const db = new Dexie("friends");
db.version(1).stores({
friends: "id, name, age"
});
(async () => {
await db.friends.put({ id: 1, name: "jane", age: 78 });
await db.friends.put({ id: 2, name: "mary", age: 76 });
const someFriend = await db.friends
.orderBy('name')
.first();
console.log(someFriend);
})();
This method returns a promise that resolves to the initial item in the collection, resulting in {id: 1, name: "jane", age: 78}.
Section 1.4: Accessing All Keys
To retrieve all keys in a collection, the keys method can be utilized:
const db = new Dexie("friends");
db.version(1).stores({
friends: "id, name, age"
});
(async () => {
await db.friends.put({ id: 1, name: "jane", age: 78 });
await db.friends.put({ id: 2, name: "mary", age: 76 });
const someFriends = await db.friends
.orderBy('name')
.keys();
console.log(someFriends);
})();
By ordering by 'name', we obtain all names stored in the database, which gives us ["jane", "mary"].
Section 1.5: Getting the Last Item
The last method allows us to fetch the last item in a collection:
const db = new Dexie("friends");
db.version(1).stores({
friends: "id, name, age"
});
(async () => {
await db.friends.put({ id: 1, name: "jane", age: 78 });
await db.friends.put({ id: 2, name: "mary", age: 76 });
const someFriends = await db.friends
.orderBy('name')
.last();
console.log(someFriends);
})();
After sorting by name, calling last retrieves the final item, yielding {id: 2, name: "mary", age: 76} in the console.
Conclusion
With Dexie, manipulating items in IndexedDB collections is straightforward. You can iterate, filter, and retrieve specific values with ease, enhancing your web application's data handling capabilities.
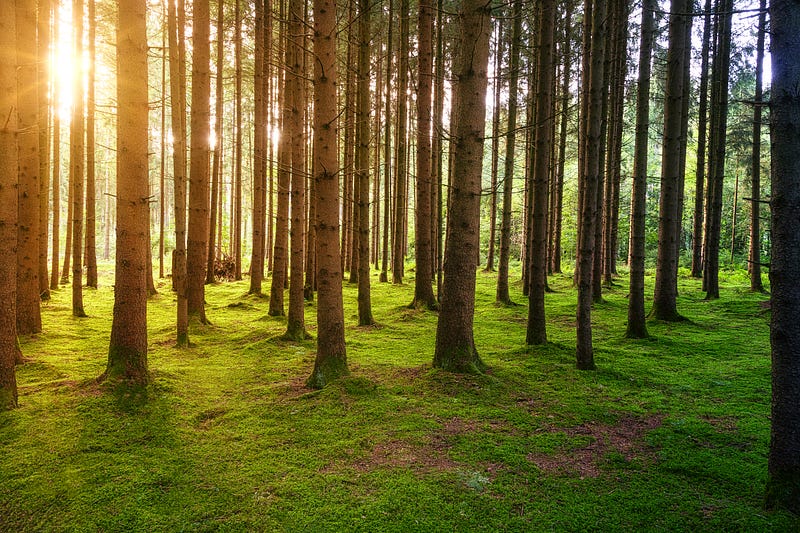