Understanding the Week Number of a Date in JavaScript
Written on
Chapter 1: Introduction to Week Numbers
When working with dates in JavaScript, you might find yourself needing to determine which week of the year corresponds to a specific date. In this article, we will explore how to achieve this in JavaScript.
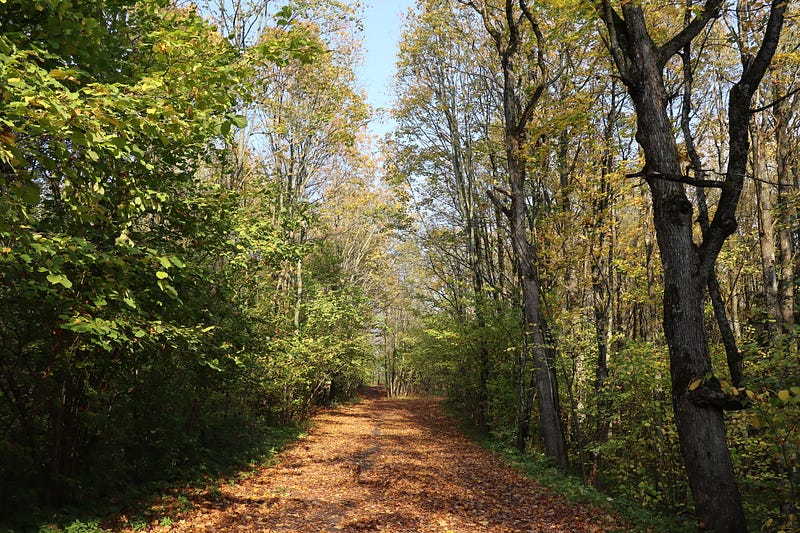
Photo by Ekaterina Tishkina on Unsplash
Section 1.1: Using Native JavaScript Date Methods
One method to find the week of the year for a specific date is by leveraging JavaScript's built-in date functions to manually calculate it. Here’s how you can do it:
const now = new Date(2021, 3, 1);
const onejan = new Date(now.getFullYear(), 0, 1);
const week = Math.ceil((((now.getTime() - onejan.getTime()) / 86400000) + onejan.getDay() + 1) / 7));
console.log(week);
In this example, we start with the date we want to analyze, referred to as now. We create another date, onejan, representing January 1 of the same year. The week number is calculated by first determining the difference in time between now and onejan, converting that into days, and then adjusting for the day of the week before dividing by 7 to get the number of weeks. Finally, we round the result up using Math.ceil, resulting in a week number of 14.
Section 1.2: Utilizing Moment.js
For a more straightforward approach, you can utilize the Moment.js library. This simplifies the process significantly:
const now = new Date(2021, 3, 1);
const week = moment(now).format('W');
console.log(week);
Here, we create a moment object by passing in our date and then use the .format('W') method to extract the week number. This method rounds down the result, giving us a week number of 13.
Chapter 2: Conclusion
In summary, you can determine the week of the year for a given date using either native JavaScript methods or the Moment.js library. Both approaches are effective, and your choice may depend on your specific needs.
Explore how to get the day of the year for a date in JavaScript with this informative video.
Watch this quick guide to understand JavaScript DATE objects in just 8 minutes!
Stay updated with more content at PlainEnglish.io. Sign up for our free weekly newsletter and connect with us on Twitter, LinkedIn, and Discord.